WordPress: A Deep Technical Approach
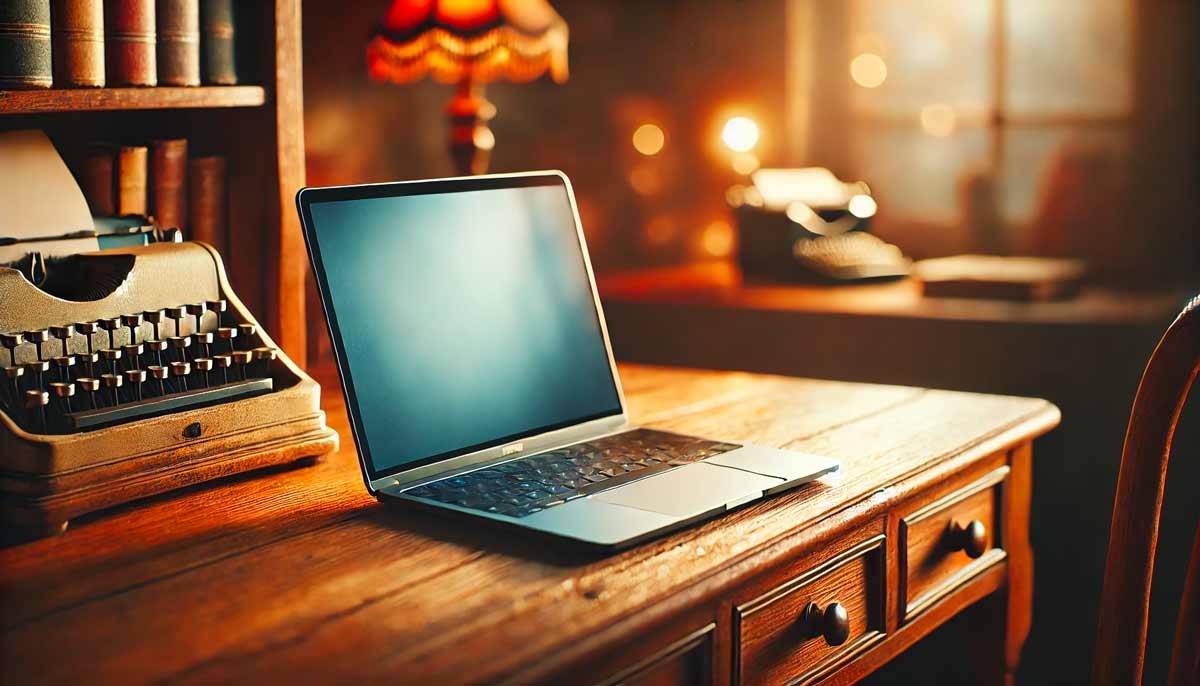
WordPress is one of the most widely used CMS (Content Management System) in the world, used to create websites of all kinds, from personal blogs to advanced e-commerce platforms. In this article, we will analyze WordPress from a technical perspective, exploring its architecture, available APIs, database structure, employed technologies, and design patterns.
1. Technology Used
WordPress is built on established and open-source technologies, including:
- Programming language: PHP (currently supports PHP 7.4, 8.0, and later)
- Database: MySQL or MariaDB
- Web Server: Apache or Nginx
- Frontend: HTML, CSS, JavaScript (with increasing use of React)
From an architectural perspective, WordPress follows a monolithic model, with backend and frontend tightly integrated, although the introduction of REST API has facilitated headless architectures.
2. Database Structure
WordPress uses a relational database, MySQL or MariaDB, with a predefined tabular structure. The main tables include:
- wp_posts: Contains posts, pages, and any other type of custom content (CPT – Custom Post Types).
- wp_postmeta: Stores metadata for posts.
- wp_users: Stores registered users.
- wp_usermeta: Contains metadata associated with users.
- wp_terms, wp_term_taxonomy, wp_term_relationships: Manage taxonomies and relationships between content.
- wp_options: Contains site configuration options.
- wp_comments, wp_commentmeta: Manage comments and related metadata.
3. WordPress API
WordPress provides various APIs to extend its functionality and integrate it with other applications:
3.1. REST API
Allows interaction with WordPress in headless mode or integration with external applications.
Example of a GET request to retrieve published posts:
curl -X GET "https://example.com/wp-json/wp/v2/posts"
3.2. Plugin API
WordPress allows developers to extend its functionality using plugins, leveraging hooks and filters.
Example of an action hook to add code during WordPress initialization:
add_action('init', function() {
error_log('WordPress has been initialized!');
});
3.3. Database API
Allows secure direct interaction with the database using the wpdb
class.
Example of a query to retrieve the latest published posts:
global $wpdb;
$results = $wpdb->get_results("SELECT * FROM {$wpdb->posts} WHERE post_status = 'publish' ORDER BY post_date DESC");
3.4. Options API
Provides a system to store and retrieve site configuration options.
Example of saving and retrieving a custom option:
update_option('my_custom_option', 'custom_value');
$value = get_option('my_custom_option');
4. Design Patterns Used in WordPress
WordPress follows several well-known design patterns:
- Singleton Pattern: Used in various core classes to ensure a single instance per component.
Example of Singleton in a plugin:
class MyPlugin {
private static $instance = null;
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new self();
}
return self::$instance;
}
private function __construct() {}
}
$plugin = MyPlugin::getInstance();
- Observer Pattern (Hooks and Filters): WordPress’s hook system follows the Observer pattern, allowing functionalities to be dynamically attached and removed.
- Factory Pattern: Used to create objects in a standardized way, such as in themes with
WP_Widget_Factory
. - MVC (Model-View-Controller) – Partial: Although WordPress does not follow a pure MVC implementation, the concept is applied in some aspects, such as themes and plugins.
5. Conclusion
Despite its monolithic architecture, WordPress offers extreme flexibility thanks to its APIs and adopted development patterns. With the evolution towards headless architectures and integration with modern technologies like React and GraphQL, WordPress remains a solid choice for web development, ensuring scalability and advanced customization.