Object-Oriented Programming (OOP) in Symfony and Laravel Frameworks
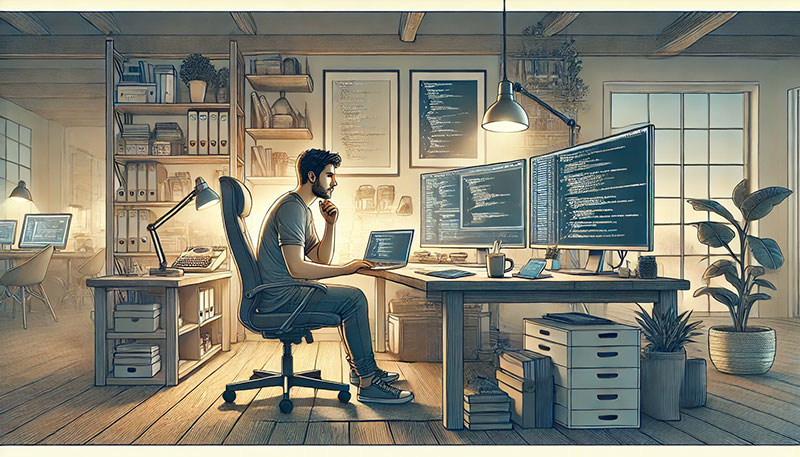
The Object-Oriented Programming (OOP) is a fundamental programming paradigm in modern PHP frameworks like Symfony and Laravel. Both use design patterns to ensure scalable, readable, and maintainable code. In this article, we will explore the main patterns used in both frameworks, comparing their implementations.
Main Design Patterns in Symfony
1. Dependency Injection (DI)
Symfony is renowned for its Dependency Injection Container (DIC). This pattern allows dependencies between classes to be decoupled, improving testability and flexibility.
- Advantage: Easy to mock dependencies in unit tests.
- Example: Services are defined in the
services.yaml
file.
2. Event Dispatcher
Symfony implements the Observer pattern through the Event Dispatcher. It allows listening to and responding to specific events in the application lifecycle.
- Advantage: Decoupling between components.
- Example: Event listeners like
kernel.request
.
3. Strategy Pattern
Symfony uses the Strategy Pattern to implement interchangeable algorithms.
- Advantage: Dynamic change of behaviors.
- Example: Authentication using different strategies (LDAP, Database, etc.).
4. Repository Pattern
Symfony uses repositories for abstract data access.
- Advantage: Separation of business logic and data access.
- Example: Repositories defined in Doctrine entities.
Main Design Patterns in Laravel
1. Service Container (Dependency Injection)
Laravel implements DI through the Service Container, similar to Symfony’s DIC.
- Advantage: Easy management of dependencies.
- Example: Dependency registration in
AppServiceProvider
.
2. Facades
Laravel uses the Facade pattern to provide a static interface to services.
- Advantage: Simple access to services.
- Example:
Cache::get('key')
.
3. Repository Pattern
Like Symfony, Laravel also implements the Repository Pattern.
- Advantage: Abstract data access logic.
- Example: Custom repositories for Eloquent models.
4. Observer Pattern
Laravel supports the Observer Pattern to monitor changes in models.
- Advantage: Decoupling event management logic.
- Example: Observers defined in Eloquent models.
Comparison Table of Symfony vs Laravel Patterns
Design Pattern | Symfony | Laravel |
---|---|---|
Dependency Injection | Dependency Injection Container | Service Container |
Observer Pattern | Event Dispatcher | Observer in models |
Repository Pattern | Doctrine Repository | Eloquent Repository |
Strategy Pattern | Authentication strategies | Not natively supported |
Facade Pattern | Not natively supported | Facades |
Conclusion
Symfony and Laravel, while sharing many design patterns, implement them in different ways. Symfony leans towards explicit configuration and flexibility, whereas Laravel focuses on rapid development with simple interfaces.