Laravel Vs Symfony – Key Concepts and Terminology
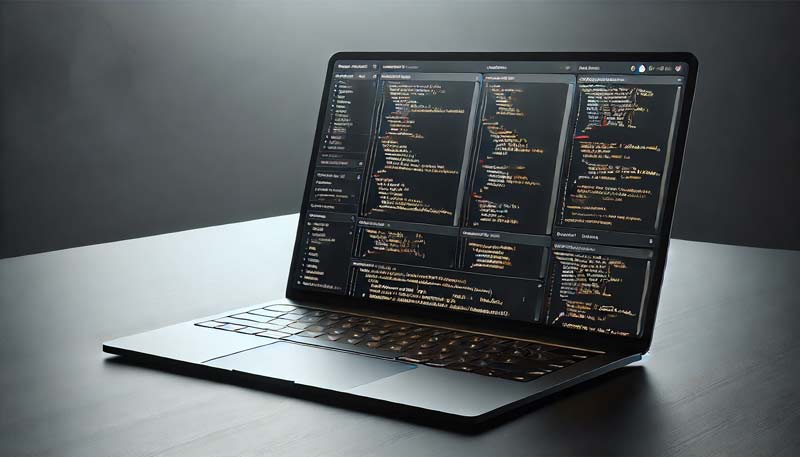
Symfony and Laravel are two very popular PHP frameworks, each with its own approach and terminology. In this article, I compare the main concepts of Laravel with their equivalents in Symfony, providing code examples for each case.
Middleware (Laravel) vs Event Subscriber (Symfony)
Laravel: Middleware
Middleware in Laravel are classes that filter HTTP requests. They can be used for tasks like authentication or logging.
<?php
namespace App\Http\Middleware;
use Closure;
class CheckAge
{
public function handle($request, Closure $next)
{
if ($request->age < 18) {
return redirect('home');
}
return $next($request);
}
}
Symfony: Event Subscriber
In Symfony, the similar concept is implemented with Event Subscribers or Kernel Events, which allow you to manipulate requests and responses.
<?php
namespace App\EventSubscriber;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Symfony\Component\HttpKernel\Event\RequestEvent;
class CheckAgeSubscriber implements EventSubscriberInterface
{
public static function getSubscribedEvents()
{
return [
RequestEvent::class => 'onKernelRequest',
];
}
public function onKernelRequest(RequestEvent $event)
{
$request = $event->getRequest();
if ($request->get('age') < 18) {
$event->setResponse(new RedirectResponse('/home'));
}
}
}
Eloquent ORM (Laravel) vs Doctrine ORM (Symfony)
Laravel: Eloquent ORM
Laravel uses Eloquent as an ORM to interact with the database through Active Record.
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = ['name', 'email', 'password'];
}
// Query example
$users = User::where('active', true)->get();
Symfony: Doctrine ORM
Symfony uses Doctrine as an ORM, based on the Data Mapper pattern.
<?php
namespace App\Entity;
use Doctrine\ORM\Mapping as ORM;
#[ORM\Entity]
class User
{
#[ORM\Id]
#[ORM\GeneratedValue]
#[ORM\Column(type: 'integer')]
private $id;
#[ORM\Column(type: 'string')]
private $name;
#[ORM\Column(type: 'string')]
private $email;
// Getters and setters...
}
// Query example
$users = $entityManager->getRepository(User::class)->findBy(['active' => true]);
Routes: Laravel vs Symfony
Laravel: Routes
In Laravel, routes are defined in files like web.php
or api.php
.
use App\Http\Controllers\UserController;
Route::get('/users', [UserController::class, 'index']);
namespace App\Controller;
use Symfony\Component\Routing\Annotation\Route;
class UserController
{
#[Route('/users', name: 'user_index')]
public function index()
{
// ...
}
}
Policies (Laravel) vs Voter (Symfony)
Laravel: Policies
Policies in Laravel are used to manage user authentication.
<?php
namespace App\Policies;
use App\Models\User;
use App\Models\Post;
class PostPolicy
{
/**
* Determine if the given post can be updated by the user.
*
* @param \App\Models\User $user
* @param \App\Models\Post $post
* @return bool
*/
public function update(User $user, Post $post): bool
{
return $user->id === $post->user_id;
}
}
Symfony: Voter
In Symfony, the equivalent concept is implemented through Voters.
<?php
namespace App\Security\Voter;
use Symfony\Component\Security\Core\Authorization\Voter\Voter;
use Symfony\Component\Security\Core\Authentication\Token\TokenInterface;
use Symfony\Component\Security\Core\Authorization\Voter\Attribute\Post;
class PostVoter extends Voter
{
protected function supports(string $attribute, $subject): bool
{
return $attribute === 'POST_EDIT' && $subject instanceof Post;
}
protected function voteOnAttribute(string $attribute, $subject, TokenInterface $token): bool
{
$user = $token->getUser();
return $user->getId() === $subject->getUserId();
}
}
Providers (Laravel) vs Symfony Services
Laravel: Providers
Service Providers in Laravel are used to register services within the application. Each provider extends the ServiceProvider
class and contains methods to configure the services.
<?php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->bind('App\Contracts\ExampleContract', function ($app) {
return new ExampleImplementation();
});
}
public function boot()
{
// Code to bootstrap services...
}
}
Symfony: Services
In Symfony, the equivalent of Providers is the Dependency Injection system through the Service Container. Services are defined in configuration files (e.g., YAML or XML) or using attributes in the class.
# config/services.yaml
services:
App\Service\ExampleService:
arguments:
$dependency: '@App\Service\DependencyService'
<?php
namespace App\Service;
class ExampleService
{
private $dependency;
public function __construct(DependencyService $dependency)
{
$this->dependency = $dependency;
}
public function doSomething()
{
// ...
}
}
Email Sending: Laravel vs Symfony
Laravel: Email Sending
Laravel uses simple syntax to send emails with built-in support for mail drivers.
Mail::to('user @ example.com')->send(new WelcomeEmail($user));
Symfony: Email Sending
Symfony uses the Mailer component.
use Symfony\Component\Mailer\MailerInterface;
use Symfony\Component\Mime\Email;
$email = (new Email())
->from('hello @ example.com')
->to('user @ example.com')
->subject('Welcome!')
->text('Hello and welcome!');
$mailer->send($email);
Conclusion
Laravel and Symfony offer powerful tools for building web applications but differ in approach and terminology. Choosing the right framework depends on the specific needs of the project and the team’s preferences.